Bionic Chess
INTRODUCTION
The world is on a collision course with a transhumanist singularity and I don’t plan to just sit back and see what happens. I’ve developed a cybernetic interface to compete for dominance with our ever approaching AI overlords…
OVERVIEW
I competed in my first ever hackathon at CU Local Hack Day 2019. I made a chess game where user input is handled with a barcode scanner and a sheet of barcodes that represent the chess board.
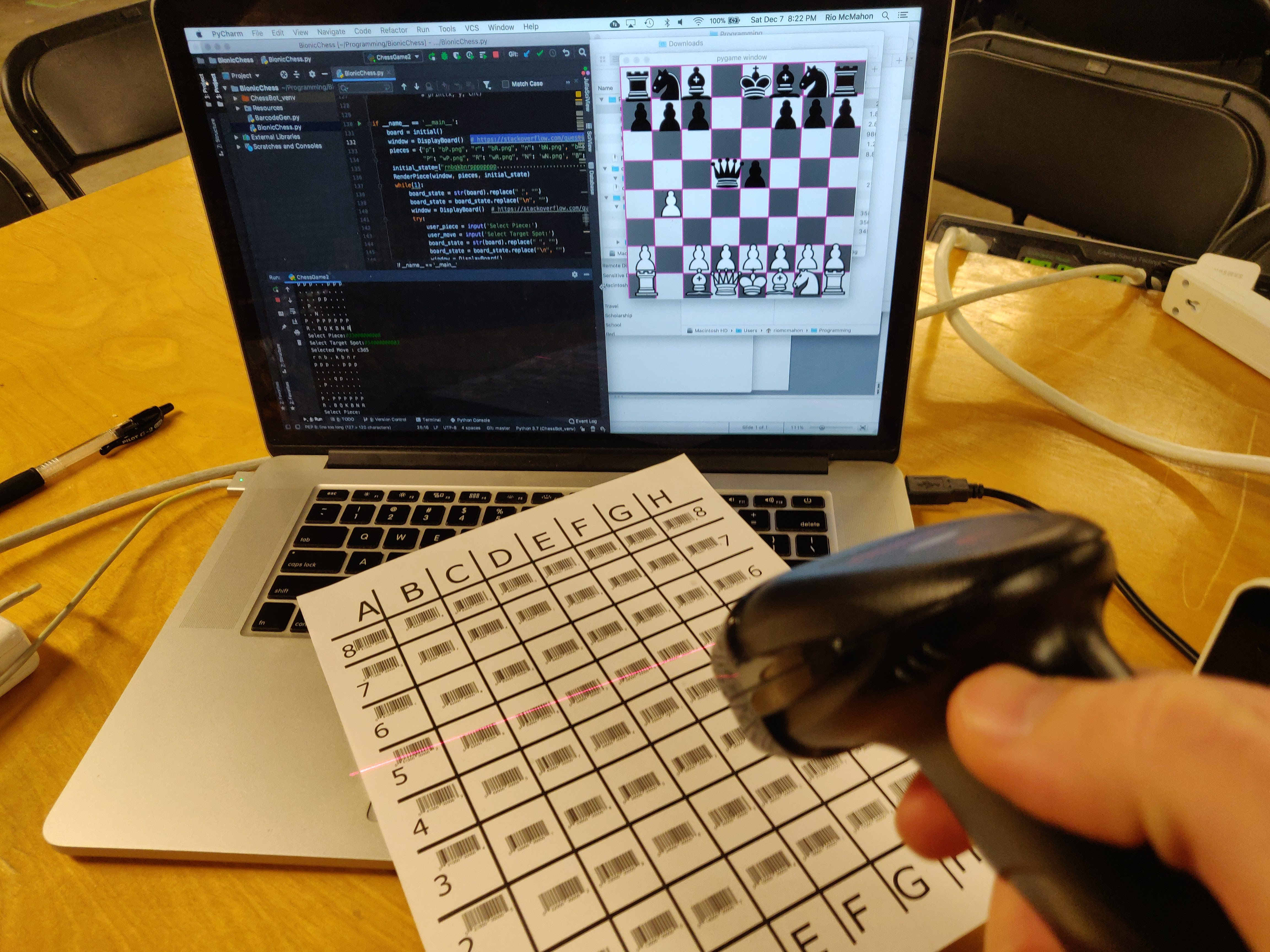
Cybernetically Augmented Gameplay
THE GAME
I used several python packages to complete the project including
As well as several other packages like pygame, etc.
The game requests user input in the form of a barcode scanner, which through trial and error I ended up implementing
using UPC-A barcodes. All the barcodes are in a format similar to 0 [R][C]000 00000 [n]
where R
is the row on the chess board (1 ≤ R
≤ 8) and C
is the column on the chess board corresponding to
the appropriate letter in the alphabet (“A” ≤ C
≤ “H”). The user scans two inputs: the piece they would like to move, and the location they’d like to move to.
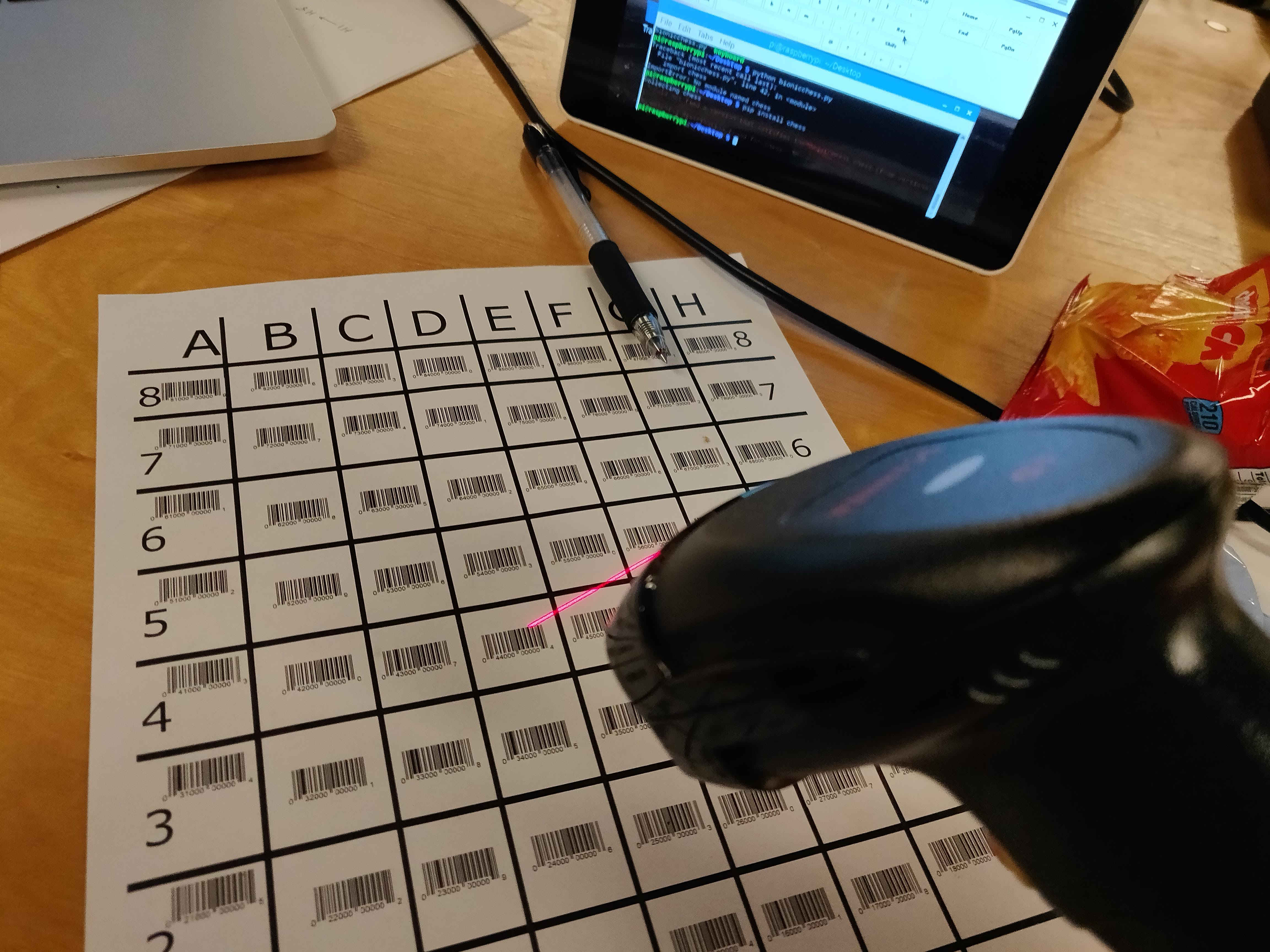
Elegant and Intuitive Human/Computer Interaction
The program handles the input and compares it to the game state which is delivered by python-chess.
|
|
which returns several useful properties about the state of the board including piece position and legal moves. If the users selected move is not legal, the program will return a notification the move is illegal. If the move is legal, the game state will update and the program will call the chess engine (Stockfish) for the computers response. Once the game state is updated again, the process repeats and chess is being played.
The game state is stored in an ASCII string
|
|
|
|
where each there are 64 positions represented by upper/lower case letters (black/white pieces) and periods (blank positions). To graphically
handle this, I looped through the 64 character string and compared the position characters to a dictionary with chess
pieces corresponding to the file location of the image of their associated game sprite. Each increment through the game state string would also change the positioning
on the game board of the pieces by xinc
and yinc
|
|
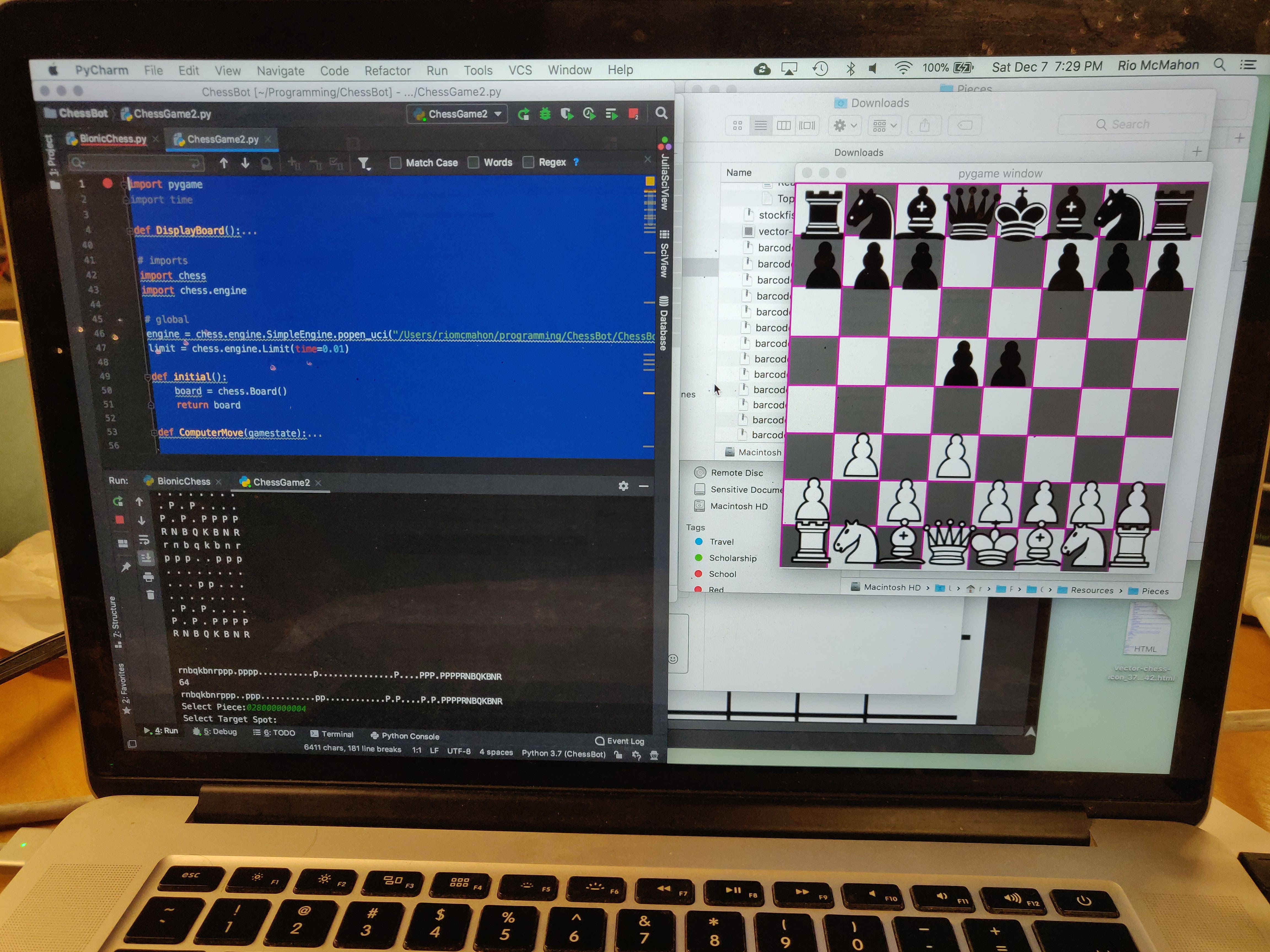
ASCII to Graphics